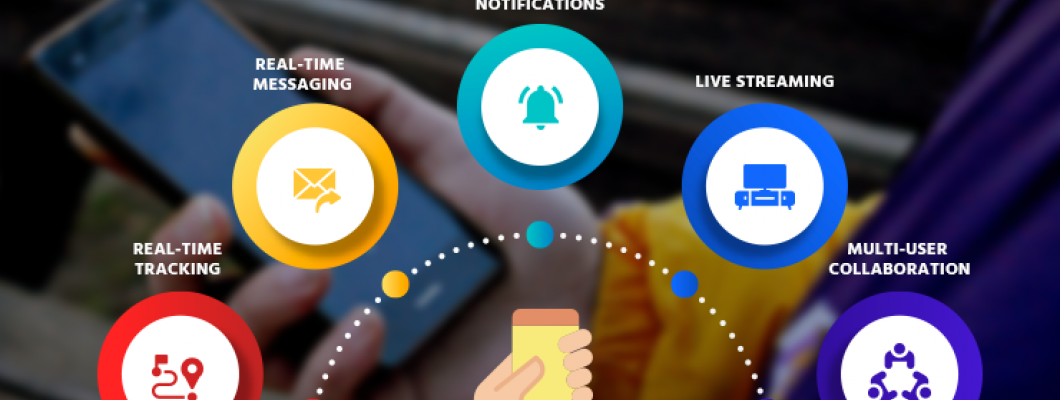
The Essentials of Real-Time Web Apps: A Beginner's Approach to Implementation
In the modern web development landscape, real-time features are no longer just an added benefit—they’re often essential for creating engaging, dynamic applications. Whether it's real-time notifications, live chat, collaborative editing, or live data feeds, real-time functionalities enhance user experience by providing instant feedback and updates. This guide will delve into the fundamentals of implementing real-time features in web applications, focusing on key technologies, best practices, and practical examples.
Table of Contents
- Understanding Real-Time Web Applications
- Key Technologies for Real-Time Features
- Choosing the Right Technology
- Implementing WebSockets in a Web App
- Using Server-Sent Events (SSE)
- Long Polling for Real-Time Updates
- Building a Real-Time Chat Application
- Best Practices for Real-Time Features
- Future Trends in Real-Time Web Development
- Conclusion
1. Understanding Real-Time Web Applications
Real-time web applications are designed to provide immediate data updates to users without requiring them to refresh their browsers. This instant communication allows for seamless interactions and enhanced user experiences.
Use Cases
- Social Media: Instant notifications for likes, comments, and messages.
- Collaboration Tools: Live editing features in applications like Google Docs.
- Gaming: Real-time multiplayer interactions.
- Financial Services: Live stock updates and market data.
- Customer Support: Chat applications for instant communication.
2. Key Technologies for Real-Time Features
There are several technologies available for implementing real-time features. Understanding these options is crucial for selecting the best fit for your project.
- WebSockets: WebSockets provide a full-duplex communication channel over a single TCP connection. This protocol allows for two-way communication between the client and server, enabling the server to push updates to clients as soon as data changes. Low Latency: WebSockets offer a faster connection compared to traditional HTTP requests. Bidirectional Communication: Both the server and client can send messages independently.
- Server-Sent Events (SSE): Server-Sent Events (SSE) is a one-way communication protocol that allows servers to push updates to clients over a single HTTP connection. Simplicity: SSE is easier to implement than WebSockets for scenarios requiring one-way communication. Automatic Reconnection: Clients can automatically reconnect if the connection is lost.
- Long Polling: Long polling is a technique where the client sends a request to the server, and the server holds the request open until new data is available. Once data is sent, the client immediately sends a new request. Compatibility: Works well with HTTP/1.1 and older systems that don’t support WebSockets or SSE. Fallback Mechanism: Can serve as a fallback option when other methods are unavailable.
3. Choosing the Right Technology
Pros and Cons of Each Approach
Feature | WebSockets | Server-Sent Events | Long Polling |
---|---|---|---|
Communication | Bidirectional | Unidirectional | Unidirectional |
Performance | Low latency | Moderate latency | Higher latency |
Complexity | More complex implementation | Simple to implement | Relatively simple |
Use Cases | Chats, gaming, collaboration | Live notifications, feeds | Polling for updates |
Performance Considerations
- WebSockets are optimal for applications requiring real-time updates with low latency.
- SSE works best for applications needing simple, continuous updates.
- Long Polling is useful when the other technologies cannot be implemented but may lead to higher latency and resource usage.
4. Implementing WebSockets in a Web App
- Setting Up a WebSocket Server
- Creating a WebSocket Client
- Handling Messages
5. Using Server-Sent Events (SSE)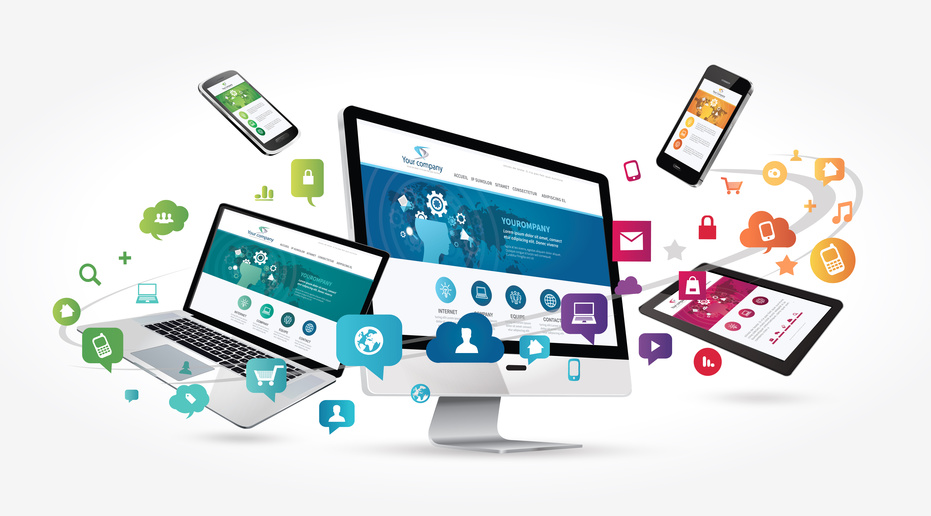
Setting Up SSE
const express = require('express');
const app = express();
app.get('/events', (req, res) => {
res.setHeader('Content-Type', 'text/event-stream');
res.setHeader('Cache-Control', 'no-cache');
res.setHeader('Connection', 'keep-alive');
setInterval(() => {
const data = JSON.stringify({ message: 'Hello from server', timestamp: new Date() });
res.write(`data: ${data}\n\n`);
}, 1000);
});
app.listen(3000, () => {
console.log('SSE server is running on http://localhost:3000/events');
});
- Easy to implement for unidirectional communication.
- Good for applications where updates are only sent from the server.
- Cannot send messages from the client to the server.
- Less efficient than WebSockets for bi-directional communication.
6. Long Polling for Real-Time Updates
How Long Polling Works
Long polling involves the client sending a request to the server, and the server keeping the request open until there is new information to send back. After receiving the response, the client immediately sends another request.
Implementing Long Polling
const express = require('express');
const app = express();
let clients = [];
app.get('/poll', (req, res) => {
clients.push(res);
req.on('close', () => {
clients = clients.filter(client => client !== res);
});
});
// Simulate data update every few seconds
setInterval(() => {
const message = JSON.stringify({ message: 'New update available!' });
clients.forEach(client => client.json({ data: message }));
clients = [];
}, 5000);
app.listen(4000, () => {
console.log('Long polling server running on http://localhost:4000/poll');
});
- You need to support older browsers that do not support WebSockets or SSE.
- The application requires a server to control when to send updates.
7. Building a Real-Time Chat Application
Overview of the Application
In this section, we’ll combine what we’ve learned to create a simple real-time chat application using WebSockets.
Backend Implementation
const WebSocket = require('ws');
const server = new WebSocket.Server({ port: 8080 });
server.on('connection', (socket) => {
socket.on('message', (message) => {
// Broadcast message to all clients
server.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
});
8. Best Practices for Real-Time Features
- Scalability: When implementing real-time features, consider how your application will scale. Using load balancers and distributing WebSocket connections across multiple servers can help manage high traffic.
- Security: Ensure that your real-time communication is secure. Use HTTPS for WebSocket connections and implement authentication to protect user data.
- Error Handling: Implement robust error handling for real-time connections. Handle cases where connections may drop and provide users with feedback.
Leave a Comment